Why Pretty Grid ?
Creating 2D or 3D grids involves a lot of boiler plate code. Especially if you want to select or manipulate different areas across your grid.
Pretty-Grid let's you define a 2D or 3D grid in a single line of code.
Additionally, Pretty-grid provides numerous helper methods to make complex point selections and transformations across multiple grids a breeze.
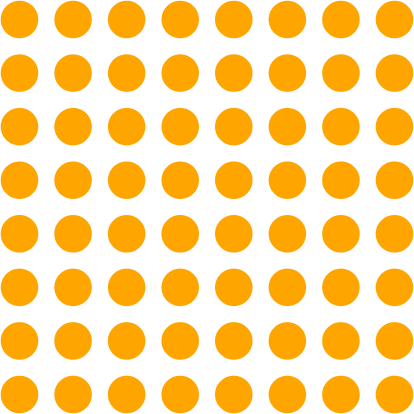
Define a grid in 1 line of code
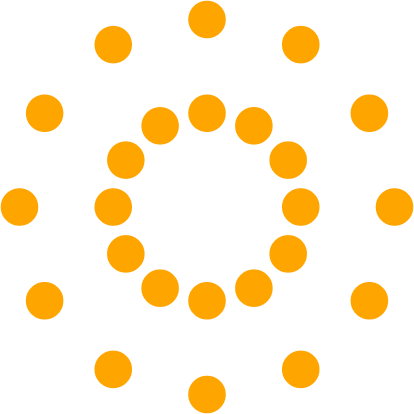
Use multiple shapes
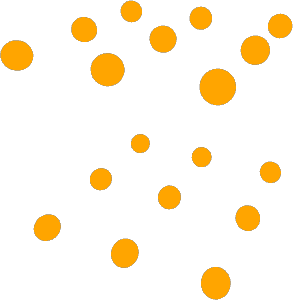
In 2 or 3 dimensions
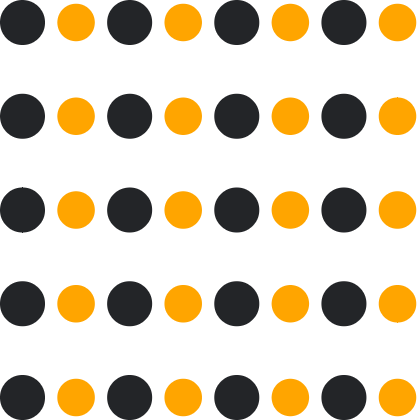
Select areas
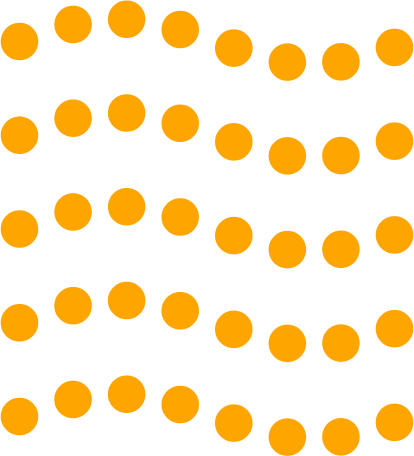
Transform points
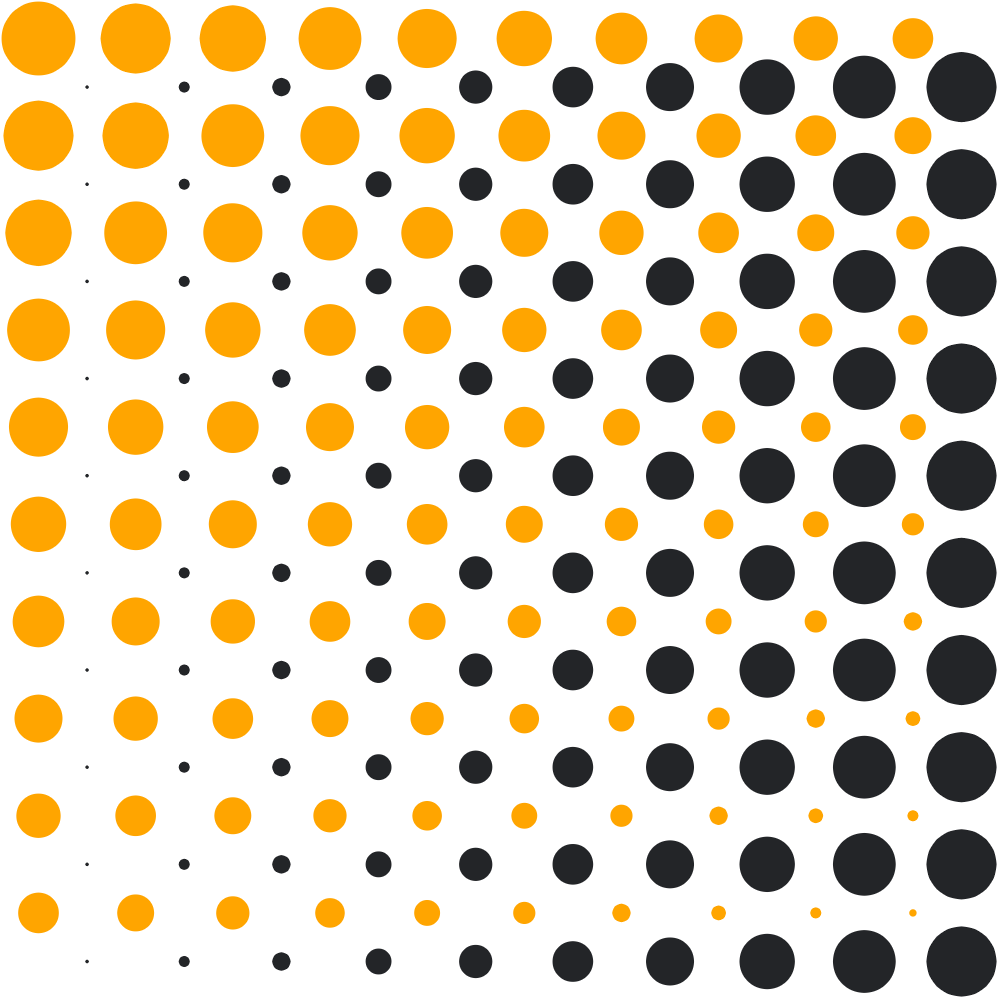
Create patterns with ease
Examples
The examples below illustrate some basic capabilities of pretty-grid
. If you want to learn more, check out the documentation section.
These examples use the powerful p5.js library to draw to the html canvas. For brevity, the examples include the
pretty-grid
code only .How to Create a 2D Grid
1 /* Creating and drawing a 2D rectangular grid */
2 import { createGrid } from "pretty-grid";
3
4 const grid = createGrid({ rows: 5, cols: 8,
5 width: 400, height: 400 });
6 grid.every(point => {
7 circle(point.x, point.y, 20);
8 };
How to Create an Elliptical Grid
1 /* Creating and drawing a 2D Ellipse grid */
2 import { createGrid } from "pretty-grid";
3
4 const grid = createGrid({ rows: 4, cols: 15,
5 width: 400, height: 400,
6 shape: GridShape.ELLIPSE
7 })
8
9 grid.every((point, _, row) => {
10 circle(point.x, point.y, (row + 1) * 5);
11 })
How to Create a 3D Grid
1 /* Creating and drawing a 3D grid*/
2import { createGrid3D } from "pretty-grid";
3
4grid = createGrid3D({ rows: 3, cols: 5, layers: 8,
5 width: 400, height: 400, depth: 400
6 })
7
How to transform points on a Grid
1 /* Creating and Transforming a 2D grid */
2import { createGrid } from "pretty-grid";
3
4const grid = createGrid({ rows: 15, cols: 15,
5 width: 400, height: 400
6 });
7
8const transformSineWave = (point) => {
9 point.x += Math.sin(point.y * 0.015) * 20;
10 return point;
11}
12
13grid.transform(transformSineWave)
14
How to draw all even/odd points on a Grid
1 /* Creating and drawing selective points on a grid*/
2import { createGrid, even, odd } from "pretty-grid";
3
4const grid = createGrid({ rows: 8, cols: 8,
5 width: 400, height: 400
6 });
7
8grid.every(point => {
9 whiteCircle(point.x, point.y);
10}, even())
11
12grid.every(point => {
13 orangeCircle(point.x, point.y);
14}, odd())
15